Exploring the Arduino UNO: A Beginner’s Guide to DIY Electronics
In this world of DIY and electronics, Arduino UNO stands out to hobbyists and professionals. Because of its simplicity and versatility. Its also very affordable to enter into Arduino and
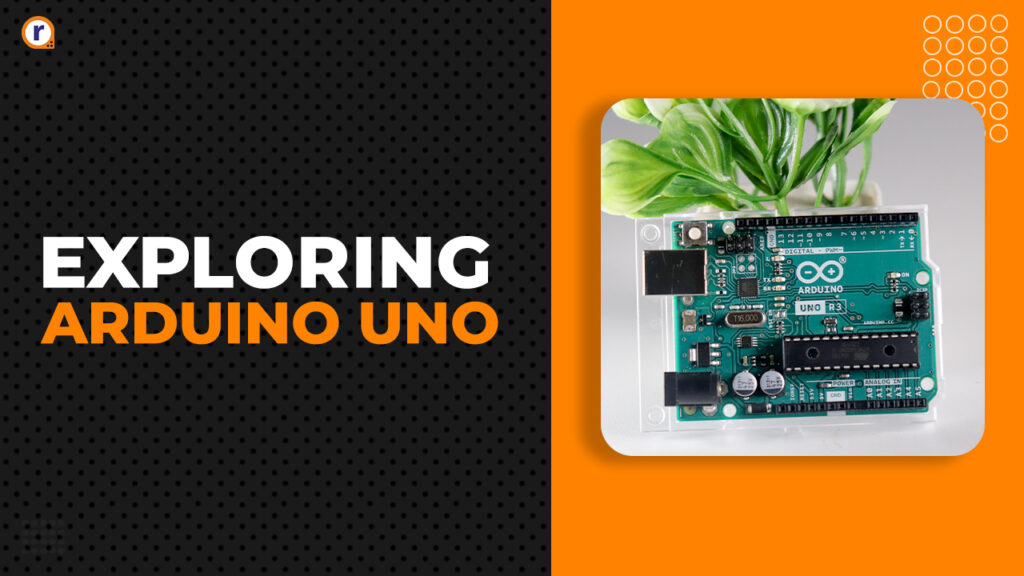
In this world of DIY and electronics, Arduino UNO stands out to hobbyists and professionals. Because of its simplicity and versatility. Its also very affordable to enter into Arduino and create something from your creative idea. It offers unlimited possibilities for experimentation and innovations.
Understanding the Arduino UNO
What is Arduino UNO? It is an open source microcontroller based on ATmega328P microcontroller. which was developed by Arduino.cc back in 2010.
The word "UNO" means one in Italian, and it was chosen to mark the major redesign of Arduino boards back then. The ATmega328 on the board comes preprogrammed with a bootloader that allows uploading new code to it without the use of an external hardware programmer.
There are many different revisions and board under Arduino. Some of them are-
- Arduino UNO R1 to R3
- Arduino R4 Minima and with Wi-Fi
- Arduino MKR boards
Arduino UNO comes with ATmega328P which is clocked at 16MHz. flash memory of 32K of which 0.5KB is taken by the bootloader. 2KB of SRAM with EEPROM of 1KB. There are few peripheral controllers on board too, such as 1x I2C, 1x SPI, 1x UART.Â
And as per connections we can make, we have 14 digital pins, 6 of them are PWM supported. 6 Analog Input pins to read data off the sensor, or to setup I2C communications.Â
As it has ATmega16U2 as USB to serial converter, we don't need FTDI converter to program it.
You can get the Original Arduino UNO R3 here -
Arduino UNO R3
Setting up Arduino UNO
To begin with Arduino UNO, you must do few things before hand. Such as downloading the Arduino IDE from their official website and install it. Install the drivers, if the board needs it.
After these, you can connect the board and write your very first blink sketch and upload it to the board and see the on board led blink.
Basics IO in Arduino UNO
Digital Pins -
To use digital pin, we need to do few things first.
1st we need to declare the pin(s) we want to use in the void setup() part.
pinMode(4, OUTPUT);
And to turn on/off the pin, we can writeÂ
digitalWrite(4, HIGH) or digitalWrite(4, LOW);
How ever there's more to some of the digital pins. We gonna come back to those later.
Analog Pins -
To use analog pins, we need to do few things first.
1st we need to declare the pins that we want to use in the void setup() part.
pinMode(A0, INPUT);
and to read of the analog pin
analogRead(A0);

Digital Pins vs Analog Pins
Digital Pins and Analog Pins have some key differences like -
Digital Pins | Analog Pins |
Can have 2 states (0v or 5 v) | Can read between 0v to 5v |
Mainly used to output related tasks | Mainly used in input related tasks |
Control LED, motor, relay and other devices | Read value of sensor and other modules |
Can be used to read( but only High and Low) | Can be used to read a range of numbers |
PWM on digital pins
What is PWM? Its Pulse Width Modulation. Basically we can turn on/off some of the output pins so fast that we can generate output of values between 0v to 5v. Why?
Lets say you want to control an LED, but you don't want it to glow it at its full brightness. Through PWM you can make it glow at any brightness level you want.Â
Not all digital pins support PWM out of the box, only pins 3, 5, 6, 9, 10, 11. We can use other pins too for PWM but using bit banging method, but this will out of scope of this guide.
To generate PWM signal at 50% brightness, we need to write like so -Â
analogWrite(9, 127);
Where the value 127 is mid value of 0 to 255 which Arduino supports.


Communications using SPI and I2C
Having all the digital, and analog pins. We have few more communication methods such as SPI and I2C. Though which we can communicate with more complex devices such as Bluetooth and Wi-Fi modules, and lot more. For example SPI can be used to connect and SD Card Module, or RFID reader you name it.
And for I2C, we can use LCD, OLED displays, sensors modules and lot more. Its especially useful as this method of communication uses only 2 wires and can support up to 127 devices given that each one of them as its own unique address using only just 2 wire.

Interrupts
Lets say if you were writing code for a project and you need to check for some event constantly. Incorporating that check in code will bog down the code's performance. So to combat this, we can set interrupt to handle those events automatically.Â
What this does is that, when a particular event occurs and it triggers the Interrupt, the Arduino halts all the process and goes to tend the Interrupt block in the code and execute that block, after executing the block it return back to were it was before that interrupt came.
A small example on how to write Interrupt -
// Pin configuration
const int buttonPin = 2; // Connect your button to digital pin 2
const int ledPin = 13; // Built-in LED on Arduino Uno
// Flag to indicate whether the LED is on or off
volatile bool ledOn = false;
void setup() {
// Initialize the button pin as input
pinMode(buttonPin, INPUT);
// Initialize the LED pin as output
pinMode(ledPin, OUTPUT);
// Enable external interrupt for digital pin 2 (interrupt 0)
attachInterrupt(digitalPinToInterrupt(buttonPin), buttonInterrupt, CHANGE);
}
void loop() {
// Main loop code can run here
// Since we're using interrupts, no need for polling the button state
}
// Interrupt service routine (ISR) for the button press
void buttonInterrupt() {
// Read the state of the button
int buttonState = digitalRead(buttonPin);
// Toggle the LED state when the button is pressed (state changes from LOW to HIGH)
if (buttonState == HIGH) {
ledOn = !ledOn;
digitalWrite(ledPin, ledOn ? HIGH : LOW);
}
}
Memory Management
Embedded systems like the Arduino Uno, which have limited resources. So, here are some tips and best practices for memory handling and writing efficient code on the Arduino Uno
-
Use Data Types Wisely:
- Choose the appropriate data type based on the range and precision required for your variables. For example, use
uint8_t
instead ofint
for variables that only need to store values from 0 to 255. - Avoid using floating-point arithmetic (
float
anddouble
) whenever possible, as they consume more memory and processing power compared to integer arithmetic (int
andlong
).
- Choose the appropriate data type based on the range and precision required for your variables. For example, use
-
Optimize Variable Usage:
- Minimize the number of global variables and prefer local variables within functions when possible. Local variables are automatically deallocated from memory when the function exits, reducing memory usage.
- Reuse variables whenever feasible instead of declaring new ones. Recycling variables can save memory and improve code readability.
-
Avoid String Manipulation:
- Manipulating strings (e.g., concatenation, parsing) consumes significant memory and processing resources on microcontrollers like the Arduino Uno.
- Instead of using the
String
class, prefer character arrays (char[]
) for storing and manipulating text data. Use functions likestrcpy()
,strcat()
, andsprintf()
for string operations.
-
Minimize Memory Fragmentation:
- Avoid dynamic memory allocation (e.g.,
malloc()
andfree()
) as much as possible, as it can lead to memory fragmentation over time. - If dynamic memory allocation is necessary, use it sparingly and deallocate memory when it's no longer needed to prevent memory leaks.
- Avoid dynamic memory allocation (e.g.,
-
Optimize Code Execution:
- Use bitwise operations (
&
,|
,<<
,>>
) for efficient manipulation of binary data instead of arithmetic operations. - Employ lookup tables for repetitive calculations or precomputed values to reduce computational overhead.
- Optimize loops and conditional statements to minimize execution time. Avoid unnecessary iterations and condition checks whenever possible.
- Use bitwise operations (
-
Enable Compiler Optimization:
- Utilize compiler optimization flags (
-O1
,-O2
,-Os
) to enable code optimization during compilation. - Compiler optimizations can reduce code size, improve execution speed, and optimize memory usage.
- Utilize compiler optimization flags (
-
Modularize Code:
- Break down complex tasks into smaller, modular functions to improve code organization and maintainability.
- Modular code allows for better reuse of code segments and facilitates debugging and troubleshooting.
-
Profile and Measure Performance:
- Use tools like the Arduino Serial Monitor, timers, and debuggers to profile code performance and identify bottlenecks.
- Profile memory usage using the
sizeof()
function and monitor stack and heap usage to ensure efficient memory utilization.
Conclusion
So with the above knowledge, whether you're a hobbyist tinkering in your garage or a professional prototyping the next big invention, the Arduino Uno offers a user-friendly platform for bringing electronic projects to life.
By mastering the basics and diving into more advanced topics, you can unleash your imagination and create anything your mind can conceive. So, grab your Arduino Uno, roll up your sleeves, and embark on an exciting journey into the realm of DIY electronics. Good Luck!
You can follow us on our Youtube channel, as we keep making newer content on Arduino and other boards. And get ideas from there to empower you next project.
best article