Developing Simple GUI Using “Tkinter” To Control GPIO’s Of Raspberry Pi
The tutorial,guides you to creating a simple GUI using Tkinter.

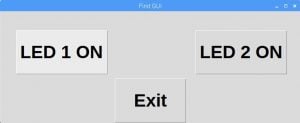
What Is A Graphical User Interface (GUI) ?
GUIÂ is a graphical desktop app that helps you to interact with the computers. Here we will be creating GUI in Raspberry Pi which will be used to interact with its GPIO. All the apps that we run on a computer are a type of GPIO.- GUI apps like Sudoku, Chess, Solitaire, etc.., are games that you can play.
- GUI apps like Chrome, Firefox, Safari, etc.., are used to surf the Internet.
What Is Tkinter ?
Tkinter is an inbuilt Python module used to create simple GUI apps. It is the most commonly used module for GUI apps in the Python. You don't need to worry about installation of the Tkinter module as it comes with Python default. Step 1 - Making connections- Make connections as shown in the diagram below.

- Here we will control Output for pins GPIO26 (Pin 37) and GPIO20 (Pin38) and will connect anode of the LED's to them.
- Pin no. 39 is used to provide ground.
Step 2 - Entering CodeÂ
- Open the python IDLE in Raspberry Pi and create a new Python file and enter the following code.
#This code has been written by Robu.in #visit https://robu.in for more information from Tkinter import * #importing Tkinter Library import tkFont import RPi.GPIO as GPIO #importing GPIO library GPIO.setmode(GPIO.BOARD) #setting up the GPIO pins GPIO.setup(37, GPIO.OUT) #setting pin37 as output pin GPIO.output(37, GPIO.LOW) #setting pin37 as LOW initially GPIO.setup(38, GPIO.OUT) #setting pin38 as output pin GPIO.output(38, GPIO.LOW) #setting pin38 as LOW initially win = Tk() #setting up window from Tk() naming win myFont = tkFont.Font(family = 'Helvetica', size = 36, weight = 'bold') #setting up font naming myfont def led1ON(): #defining function led1ON print("LED1 button pressed") #to be printed on terminal if GPIO.input(37) : GPIO.output(37,GPIO.LOW) #setting pin37 to low led1Button["text"] = "LED 1 ON" #text for led1Button else: GPIO.output(37,GPIO.HIGH) #setting pin37 to high led1Button["text"] = "LED 1 OFF" #text for led1Button def led2ON(): print("LED2 button pressed") if GPIO.input(38) : GPIO.output(38,GPIO.LOW) #setting pin38 to low led2Button["text"] = "LED 2 ON" #text for led2Button else: GPIO.output(38,GPIO.HIGH) #setting pin38 to high led2Button["text"] = "LED 2 OFF" #text for led2Button def exitProgram(): print("Exit Button pressed") GPIO.cleanup() #Quitting program win.quit() win.title("My GUI") #title for window win.geometry('800x300') #Dimensions of the window exitButton = Button(win, text = "Exit", font = myFont, command = exitProgram, height =2 , width = 6) #setting button naming exitbutton exitButton.pack(side = BOTTOM) #button position for exitbutton #commanding to button to exitProgram led1Button = Button(win, text = "LED 1 ON", font = myFont, command = led1ON, height = 2, width =8 ) #setting button naming led1Button led1Button.place(x=37, y=50) #button position for led1Button #commanding to button to led1ON function led2Button = Button(win, text = "LED 2 ON", font = myFont, command = led2ON, height = 2, width =8 ) #setting button naming led2Button led2Button.place(x=520, y=50) #button position for led2Button #commanding to button to led2ON function mainloop() #commanding mainloop for starting main loop
- Working of each command mentioned in the corresponding comment.
- Now save the program. We are saving it as gui_project python file.
- Now open terminal and type command sudo python gui_project.py and press Enter.

- Now the window naming My GUI will open up as shown.

- Now you can control the pins with this graphical user interface. If you have touch display, you can also use it by touch.
- Now you can yourself explore the world of GUI from Tkinter. For more help on tkinter visit : https://wiki.python.org/moin/TkInter
Tags : Raspberry Pi Article