Getting Started with Arduino IoT Cloud: Exchanging Data Between the Cloud and Nano ESP32
In today’s world, the Internet of Things (IoT) refers to a network where billions of physical devices are connected to the internet. Not only are there billions of devices already
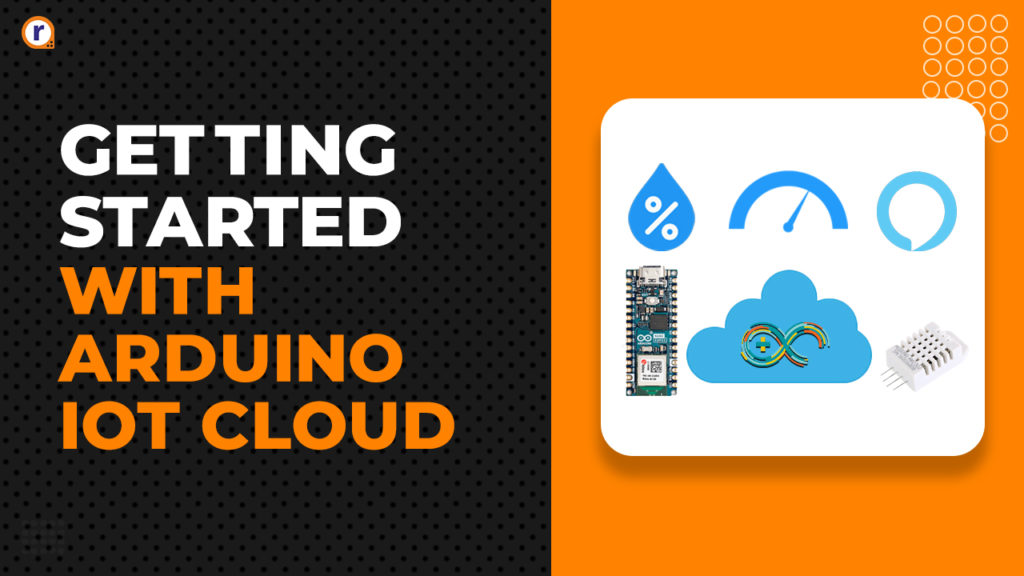
In today's world, the Internet of Things (IoT) refers to a network where billions of physical devices are connected to the internet. Not only are there billions of devices already connected, but the number of connected devices is also increasing by billions every year.
To support this vast number of IoT devices, a robust infrastructure is needed to handle the transaction of all the data. This infrastructure is provided by IoT cloud platforms such as Ubidots, IFTTT, Blynk, ThingSpeak, Particle Cloud, and others. However, in this tutorial, we will be focusing on the Arduino IoT Cloud platform. Why? You might ask. To me, the pricing is reasonable, the interface is clean, and it is feature-rich. So, without further ado, let's dive right into it.
What is the Arduino IoT cloud?
The Arduino IoT Cloud is a platform for IoT devices and applications. It facilitates connecting Arduino devices to the internet, data collection, remote control, and automation. Users can control devices, create automation and more. It is designed for ease of use, even for non-technical users.
How does Arduino IoT cloud work?
The Arduino IoT Cloud allows you to register and program your Arduino device, create a 'Thing' with properties, widgets, and actions, and then monitor, control, and automate your device from the dashboard. It also provides APIs for integration with other services.
Features of Arduino IoT Cloud
From pre-built Templates to web hook APIs there are a ton of features that the Arduino IoT cloud has to offer, all of those features are listed below.
- Data Monitoring - learn how to easily monitor your Arduino's sensor values through a dashboard.
- Variable Synchronization - variable synchronization allows you to sync variables across devices, enabling communication between devices with minimal coding.
- Scheduler - schedule jobs to go on/off for a specific amount of time (seconds, minutes, hours).
- Over-The-Air (OTA) Uploads - upload code to devices not connected to your computer.
- Webhooks - integrate your project with another service, such as IFTTT.
- Amazon Alexa Support - make your project voice controlled with the Amazon Alexa integration.
- Dashboard Sharing - share your data with other people around the world.
List of Arduino Cloud Supported Devices
To use the Arduino IoT cloud platform you'll have to have to have a cloud compatible Arduino board. For that you can choose an official Arduino board or a third party board based on the ESP32 / ESP8266 microcontroller. All the supported boards are listed below.
The following boards connect to the Arduino IoT Cloud via Wi-Fi.
- MKR 1000 Wi-Fi
- MKR Wi-Fi 1010
- Nano RP2040 Connect
- Nano 33 IoT
- GIGA R1 Wi-Fi
- Portenta H7
- Portenta H7 Lite Connected
- Portenta Machine Control
- Nicla Vision
- Opta.
Other than that the Arduino IoT Cloud also supports LoRa WAN via The Things Stack and it also supports GSM and NB-IoT boards like the MKR GSM 1400 and MKR NB 1500 other than that it also supports GSM and NB-IOT Boards like MKR GSM 1400 and it has support for ESP32 and ESP8266.
Configuring the Arduino IOT Cloud
Setting up the Arduino Cloud is easy and simple, let's take a look at how to go from start to finish!
- Creating an Arduino Account
To start using the Arduino IoT Cloud, you need to have an Arduino account. If you don't have one, you will need to create one.
- Go to the Arduino IoT Cloud
Now click on the dotted box shaped menu icon, and click on IoT Cloud. You can also go directly to the Arduino IoT Cloud, by clicking on the link.
- Creating A Thing
Now you need to create a Thing. A Thing is a connected device that can communicate with the cloud. You can make your Things interact with other Things or anything else in the physical world. To get started you need to click on the Create Thing button.
Here you need to do four things, first you need to name your Thing. Naming a thing will make it easy for you to find the device later.
Now , you need to click on the Add variable and you will be presented with the above window , where you need to give a name to the variable. We named our variable led and on the Select variable dropdown we have selected the Alexa compatible and Basic type variable. Now for the variable type we have selected light. Finally as for the Variable Permission we will select Read & Write and for the Variable Update Policy we will select on change. And we will save the variable.
The next variable is for Temperature. We will give the name for the variable and we will set the variable type as float. And we will do the same for humidity.
Now we will associate a device to do so we need to click select Device on the Associated Device section, and you need to associate a new device, but first you need to install the drivers.
In this section you will have three options, if you have an genuine Arduino board you will select the Arduino Board option and if you are using other development boards like the ESP32 or ESP8266 you have to select the third party board.
Once device setup is successful you will be presented with your Device ID and Secret Key, save that as PDF and copy the Secret key to continue further.
Once that is done you need to enter your Wi-Fi Credentials and the Secret Key and click on Save. and you are done with this part.
Now we need to click on the sketch Tab. and we will write our code here. The code is very simple and the whole code is given at the bottom of the page in the Arduino Code Section.
When you declare the variables in the setup section, the IoT cloud automatically creates the callback functions for the variable and if any changes is detected by the cloud the callback function is called and the associated action gets executed.
Building a Dashboard
Now we need to create a dashboard for our project, when the dashboard is created you can control your application with the dashboard from your browser or your Android or IoS application.
When you click on Build Dashboard you will be presented with the above interface, and you need to click on the ADD button and you need to select the switch. Through which we are going to be controlling the LED.
Now you need to assign a variable to the newly added widget. To do so click on the Link Variable button and we have to link the led variable with the switch. Once that is done will will add in a gauge and a percentage meter and attach the variable with it.
If you have done everything correctly the code should compile fine and you can see the log in the serial monitor window.
Final Code
/*
Sketch generated by the Arduino IoT Cloud Thing "Untitled"
https://create.arduino.cc/cloud/things/f2395d6c-425a-44c5-9780-e415968881b1
Arduino IoT Cloud Variables description
The following variables are automatically generated and updated when changes are made to the Thing
float humidity;
float temperature;
CloudLight led;
Variables which are marked as READ/WRITE in the Cloud Thing will also have functions
which are called when their values are changed from the Dashboard.
These functions are generated with the Thing and added at the end of this sketch.
*/
#include "thingProperties.h"
#include "DHT.h"
#define DHTPIN 5
#define DHTTYPE DHT22
DHT dht(DHTPIN, DHTTYPE);
int interval=2000;
unsigned long previousMillis=0;
void setup() {
// Initialize serial and wait for port to open:
Serial.begin(9600);
// This delay gives the chance to wait for a Serial Monitor without blocking if none is found
delay(1500);
dht.begin();
// Defined in thingProperties.h
initProperties();
// Connect to Arduino IoT Cloud
ArduinoCloud.begin(ArduinoIoTPreferredConnection);
pinMode(13,OUTPUT);
/*
The following function allows you to obtain more information
related to the state of network and IoT Cloud connection and errors
the higher number the more granular information you’ll get.
The default is 0 (only errors).
Maximum is 4
*/
setDebugMessageLevel(2);
ArduinoCloud.printDebugInfo();
}
void loop() {
ArduinoCloud.update();
// Your code here
unsigned long currentMillis = millis();
if ((unsigned long)(currentMillis - previousMillis) >= interval) {
humidity = dht.readHumidity();
temperature = dht.readTemperature();
previousMillis = currentMillis;
}
}
/*
Since Led is READ_WRITE variable, onLedChange() is
executed every time a new value is received from IoT Cloud.
*/
void onLedChange() {
(led == 1) ? digitalWrite(13,HIGH) :digitalWrite(13,LOW);
}
/*
Since Temperature is READ_WRITE variable, onTemperatureChange() is
executed every time a new value is received from IoT Cloud.
*/
void onTemperatureChange() {
// Add your code here to act upon Temperature change
}
/*
Since Humidity is READ_WRITE variable, onHumidityChange() is
executed every time a new value is received from IoT Cloud.
*/
void onHumidityChange() {
// Add your code here to act upon Humidity change
}
“Thank you for sharing such an insightful and inspiring blog post! https://www.kathirsudhirautomation.com/ “